gRPC Protocol
gRPC Protocol
Introduction
Zilla supports gRPC (Google Remote Procedure Call) as a protocol binding, designed to enable high-performance, low-latency communication between clients and services in modern distributed systems. gRPC uses HTTP/2 for transport, providing features like bidirectional streaming, flow control, and efficient binary serialization using Protocol Buffers (Protobuf). This makes gRPC ideal for microservices, real-time communication, and APIs requiring high throughput and low latency.
gRPC Request-Response Flow
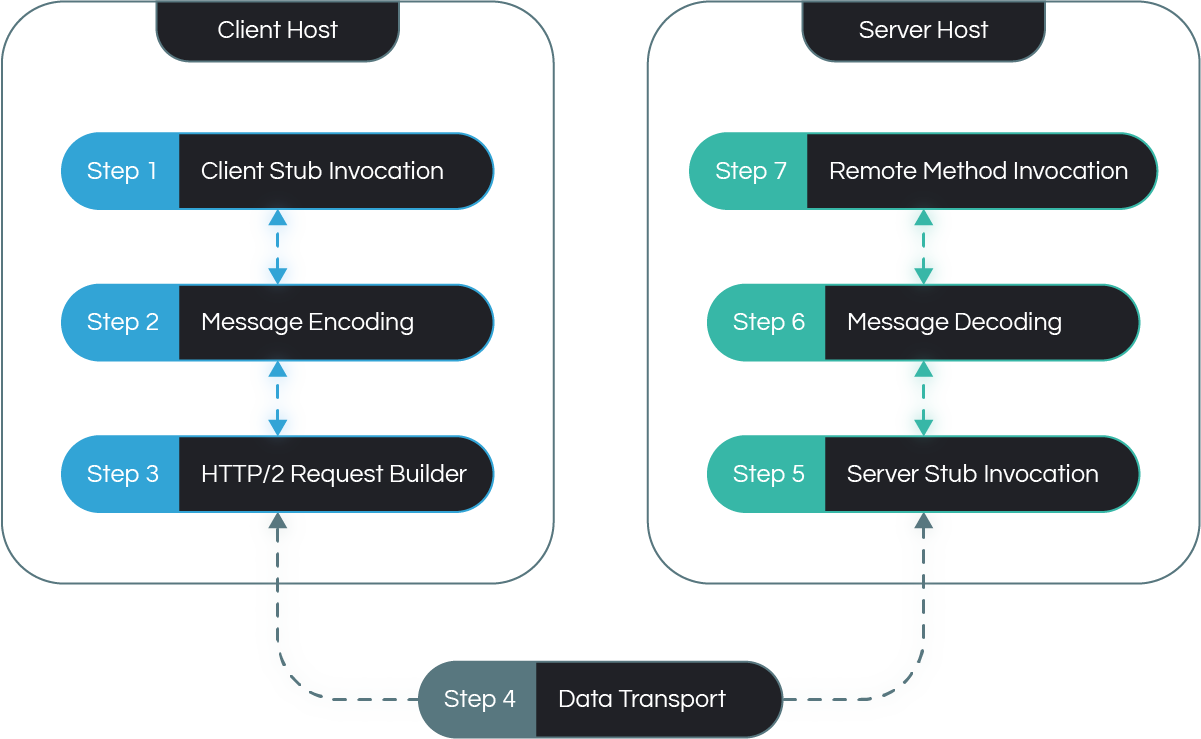
- Client Stub Invocation: The client calls a gRPC method via the client stub.
- Message Encoding: The client serializes the request using Protobuf.
- HTTP/2 Request Building: The serialized message is wrapped into an HTTP/2 request.
- Data Transport: The request is sent over the network to the server.
- Server Stub Invocation: The server receives the request and passes it to the server stub.
- Message Decoding: The server deserializes the Protobuf message.
- Remote Method Invocation: The server invokes the appropriate method and generates a response.
- Response Processing: The response follows the reverse path: encoding, HTTP/2 transport, decoding, and client processing.
gRPC Request Structure
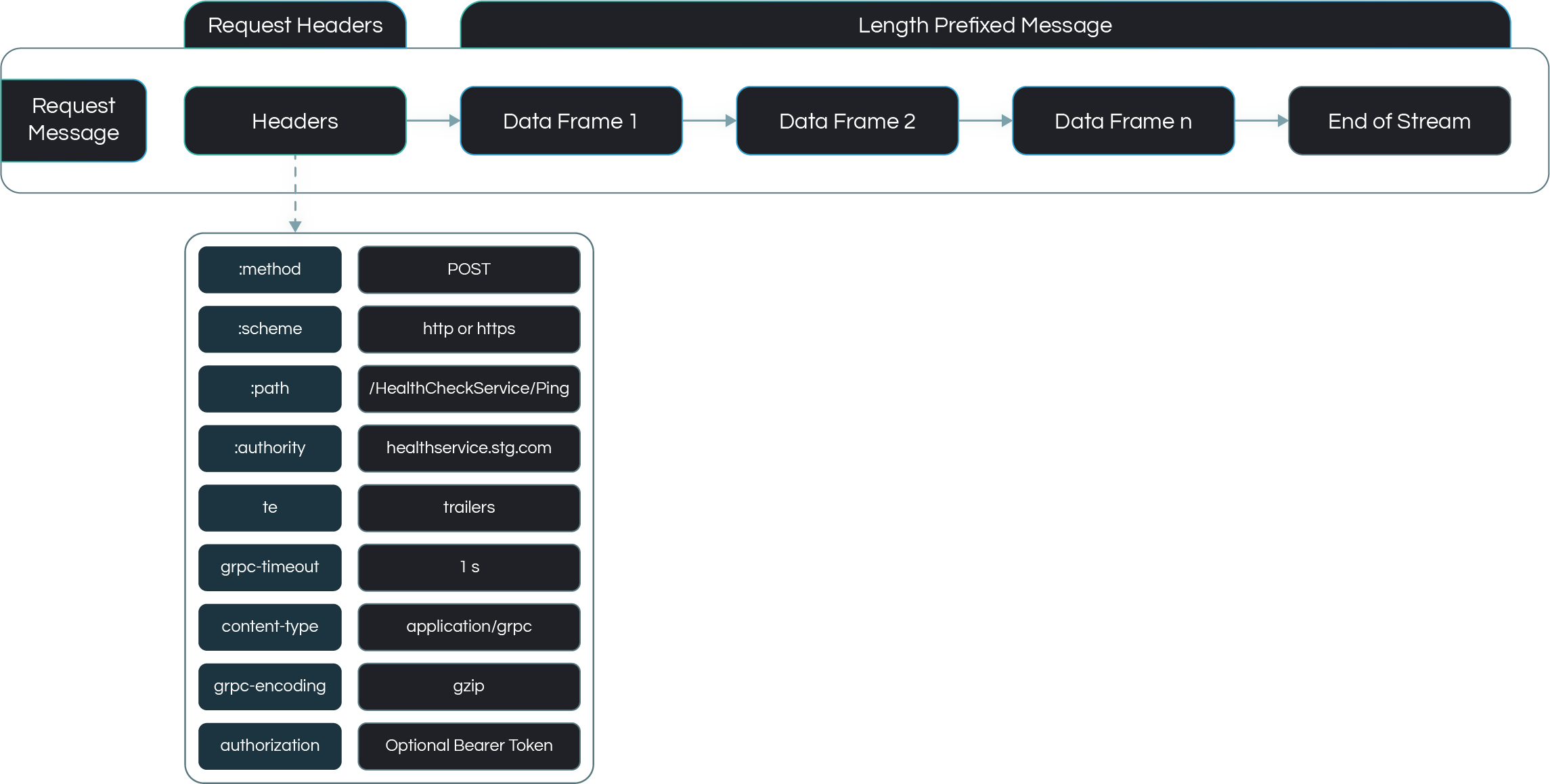
syntax = "proto3";
service Greeter {
RPC SayHello (HelloRequest) returns (HelloResponse);
}
message HelloRequest {
string name = 1;
}
message HelloResponse {
string message = 1;
}
POST /Greeter/SayHello HTTP/2
Content-Type: application/grpc
gRPC Response Structure
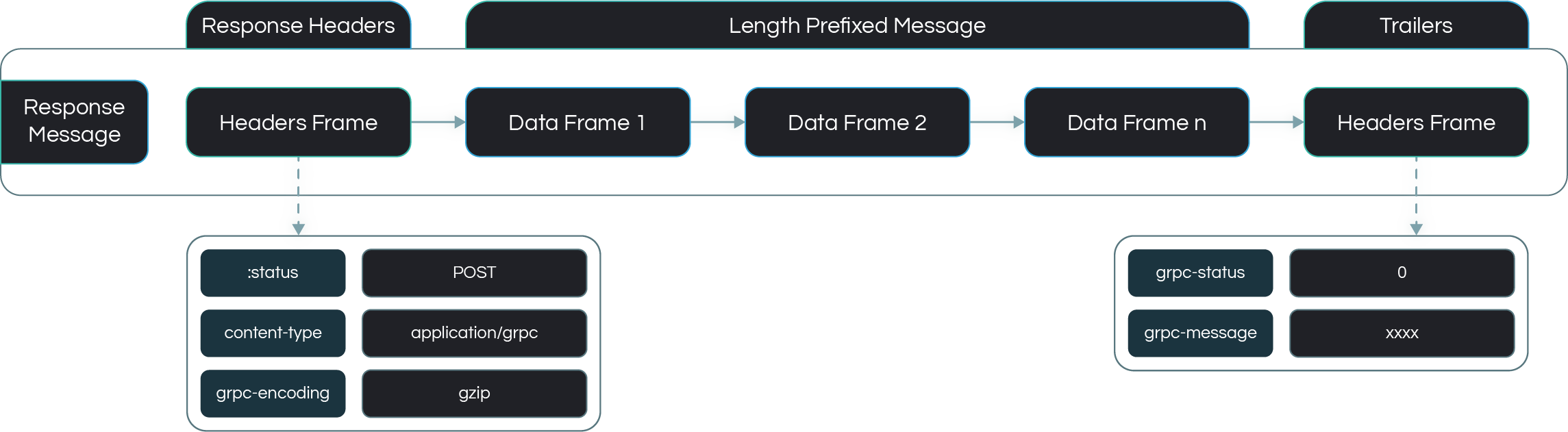
HTTP/2 200 OK
Content-Type: application/grpc
<Serialized Protobuf Payload>
Note
While it is possible to perform gRPC calls directly with HTTP, it is advised to call gRPC endpoints through generated code in the designated programming language.
Supported Features
- Unary RPC: Simple request-response model.
- Server Streaming RPC: The client sends a single request, and the server streams multiple responses.
- Client Streaming RPC: The client streams multiple requests, and the server sends a single response.
- Bidirectional Streaming RPC: Both client and server stream data simultaneously.
Comparing REST API (JSON) vs gRPC API (Protobuf)
Feature | REST API (JSON) | gRPC API (Protobuf) |
---|---|---|
Format | Human-readable test | Compact binary |
Data Size | Larger due to text-based format | More efficient due to binary encoding |
Debugging | Easily readable and editable | Require tools for decoding |
Type Safety | Not strictly enforced, relies on conventions | Strongly enforced, prevents schema violations |
Performance | Slower due to text parsing and conversion | Faster Faster with optimized serialization/deserialization |
Security
Securing gRPC with TLS
gRPC encrypt data in transit by utilizing TLS integrated with HTTP/2. Zilla provides support for TLS bindings to enforce encryption and protect gRPC communication.
Authentication
Zilla natively supports JWT-based authentication, using a guard
implementation that allows seamless validation and access control for protected resources.
JWT is a compact, URL-safe token used for authentication and authorization. It consists of three parts:
- Header: Contains the type of token (
JWT
) and the signing algorithm (HS256
orRS256
). - Payload: Contains claims (user data, expiration, roles, etc.).
- Signature: Ensures the token has not been tampered with.
During authentication, the client sends the token in the Authorization header or requests metadata with the Authorization key on each request:
Authorization: Bearer <JWT-TOKEN>
response, call = stub.SayHello.with_call(
helloworld_pb2.HelloRequest(name="you"),
metadata=(
("Authorization", "Bearer ..."),
),
)
The server validates the token’s integrity and claims before granting access.
Zilla: Beyond Standard gRPC
Zilla enhances traditional gRPC workflows by integrating advanced validation, seamless protocol bridging, and dynamic access control.
- Data Governance: Validate metadata, payloads, and request/response content with Schema Registry support.
- Protocol Mapping: Enable effortless communication with Kafka through native Kafka Wire Protocol integration.
Zilla: gRPC Use Cases
Zilla leverages gRPC Protocol to provide powerful proxying, event streaming, and secure communication.
Reference
grpc Binding The grpc
support, with server
or client
behavior.
grpc-kafka Binding The proxy
kind grpc-kafka
binding adapts gRPC request-response streams to Kafka topic streams.